Singleton Design Pattern - All that you wanted to know
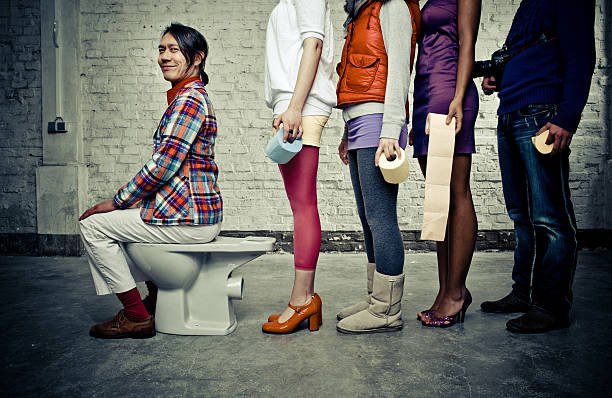
Have you ever found yourself in a situation where you need to ensure that there's only one instance of a particular object created during runtime? This is where the Singleton Design Pattern comes into play. In this blog post, we'll explore everything you need to know about the Singleton pattern, including when and why it should be used. Whether you're an experienced developer or just starting out, understanding this design pattern can prove invaluable in ensuring your code is optimized for performance and scalability. So join us as we delve into the world of the Singleton pattern!
What is the Singleton Design Pattern?
The Singleton design pattern is a software design pattern that restricts the instantiation of a class to one object. This is useful when only one instance of a class is needed to coordinate actions across the system. The Singleton pattern is also known as the Singleton anti-pattern because it can lead to code that is difficult to maintain and test.
When to Use the Singleton Design Pattern
The singleton design pattern is a powerful software engineering tool that can be used in a variety of situations. When used correctly, it can help improve the efficiency and flexibility of your code. However, like all tools, it has its own limitations and should be used with care.
In general, the singleton design pattern should be used whenever you need to ensure that only one instance of a particular class exists in your application.
- When you need a global point of access to an object, such as a thread pool or a cache.
- When you want to avoid creating multiple copies of an object, such as with a database connection pool.
- When you want to ensure that only one instance of an object can be created, such as with a window manager.
- When you want to lazy-initialize an object, meaning the object is not created until it is first needed.
- When you want to centralize control over an object's creation and lifecycle, such as with a logging service.
- if you have a class that provides access to a configuration file, you might want to use the singleton design pattern to ensure that there is only one instance of the class (and therefore only one copy of the configuration file) in your application.
The above reasons are why the Singleton design pattern is used. There are many different ways to implement the Singleton pattern, each with its own trade-offs and benefits. The most important thing to remember when using the Singleton pattern is that it should only be used when absolutely necessary, as it can make your code more difficult to maintain and test.
Of course, there are also times when you should not use the singleton design pattern. In particular, you should avoid using singletons for classes that are not thread-safe. If multiple threads try to access a non-thread-safe singleton at the same time, they may end up corrupting each other's data.
Benefits of Using the Singleton Design Pattern
Perhaps the most obvious benefit is that it ensures that only one instance of a class is created. This can be very useful when you need to manage resources in an application, such as database connections or file handles. Another benefit of using the Singleton design pattern is that it can help to promote code reuse. If you have a utility class that is used throughout your application, making it a Singleton means that it will be easy to use in any other part of the codebase.
Finally, using the Singleton design pattern can help to make your code more efficient. By ensuring that only one instance of a class is created, you can avoid the overhead associated with creating multiple instances.
How to Implement the Singleton Design Pattern in Java
The singleton design pattern is one of the most commonly used software design patterns. It is a creational design pattern that allows for the creation of only one instance of a class. This instance can be global and accessed by any other part of the code. The singleton design pattern is often used in Java programs to provide a global point of access to a resource or object.
There are many different ways to implement the singleton design pattern in Java. The most common way is to use the static keyword when declaring the instance variable. This ensures that there can only ever be one instance of the class. The static keyword also allows the instance to be accessed without having to create an object first.
Another way to implement the singleton design pattern in Java is through the use of an enum. Enums are special data types that allow for a fixed set of values. By using an enum, we can create a Singleton class that can only ever have one value.
Finally, we can also use the double-checked locking mechanism to ensure that only one instance of our Singleton class is created. Double-checked locking works by first checking if an instance exists before trying to create one. If an instance already exists, it simply returns this existing instance instead of creating a new one.
Implementing the singleton design pattern in Java is relatively simple and straightforward.
Pros and Cons of Using the Singleton Design Pattern
There are a few key considerations to take into account when deciding whether or not to use the Singleton design pattern. These include:
-Ease of implementation: The Singleton pattern is relatively easy to implement, especially in languages like Java that have built-in support for it.
-Flexibility: The Singleton pattern can be easily extended to support additional features such as lazy initialization and thread safety.
-Testability: The fact that only a single instance of a Singleton class can exist makes it easy to test and debug.
On the downside, the Singleton design pattern can lead to some issues if not used correctly, such as:
-Increased complexity: The additional flexibility offered by the Singleton pattern comes at the cost of increased complexity. This can make code more difficult to understand and maintain.
-Global state: By its very nature, the Singleton design pattern introduces a global state into an application. This can make it harder to reason about code and can lead to unexpected side effects.
Sample Code
public final class Singleton {
private static Singleton instance;
public String value;
private Singleton(String value) {
// The following code demonstrates the lazy/slow initialization.
try {
Thread.sleep(1000);
} catch (InterruptedException ex) {
ex.printStackTrace();
}
this.value = value;
}
public static Singleton getInstance(String value) {
if (instance == null) {
instance = new Singleton(value);
}
return instance;
}
}
Conclusion
The Singleton Design Pattern is a useful programming tool that can be used to ensure the same instance of an object is accessed by multiple threads. It's an important concept for software developers to understand and apply when needed, as it can help streamline complex operations and make code more efficient. With careful consideration of when and why you should use this design pattern, it's sure to greatly improve the development process.