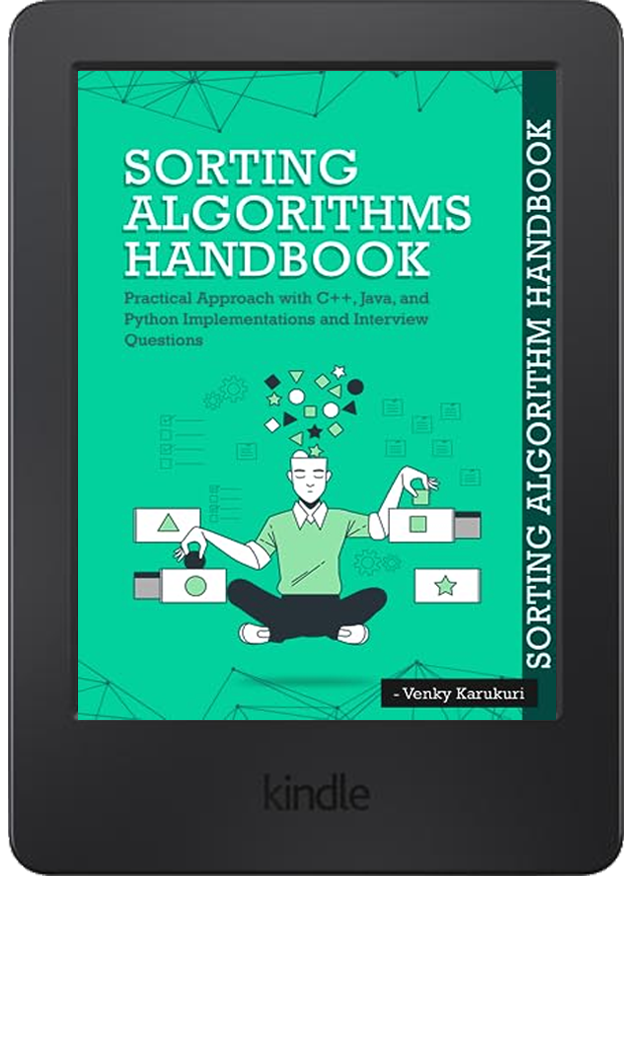
Book introduction
14 different sorting algorithms explained in detail. This is a one-stop resource for all your sorting needs. This book introduces sorting algorithms in a comprehensive way. The fundamental concepts and techniques of each sorting algorithm are presented in a clear and concise manner, with an emphasis on their practical applications.
Code strategies
Unlock the secrets of effective problem-solving in coding with 'Code Strategies.' This book reveals practical solutions to common challenges. 'Code Strategies' is your guide to crafting better, more effective code. Learn real-world applications with easy-to-follow examples and take your programming skills to the next level.
Benefits
This book will change your understanding of sorting algorithms forever and you will be able to crack interview questions related to sorting algorithms
What's inside the book
This book is designed for students and professionals with a background in Computer Science and Information Technology. This book introduces sorting algorithms in a comprehensive way. The fundamental concept and technique of each sorting algorithm are presented in a clear and concise manner, with an emphasis on their practical applications.
Simple sort
A simple, inefficient sorting algorithm that uses brute-force sorting strategy for sorting.
Bubble sort
A simple, inefficient sorting algorithm that repeatedly iterates through a list and swaps adjacent elements that are out of order.
Selection sort
An inefficient sorting algorithm that repeatedly selects the minimum element from an unsorted part of a list and places it at the end of the sorted part.
Quick sort & parallel quick sort
An efficient, production-ready sorting algorithm that follows the divide and conquer strategy by selecting a "pivot" element from a list and partitioning the other elements into two sublists according to whether they are less than or greater than the pivot.
Counting sort
An efficient, non-comparative integer sorting algorithm that counts the number of occurrences of each possible key value in an array and uses this information to sort the keys in the array <
Radix sort
An efficient, non-comparative integer sorting algorithm that sorts the elements of a list according to their digits, starting with the least significant digit.
Insertion sort
A simple, efficient sorting algorithm that builds a final sorted list one element at a time, inserting each element in its correct position in the sorted list.
Shell sort
An efficient, improvised insertion sort that allows elements to be moved further towards their final position by "hiking" them through a list, using a diminishing increment sequence.
Merge sort & parallel merge sort
An efficient, production-ready divide and conquer sorting algorithm that recursively divides a list into smaller sublists, sorts the sublists, and then merges the sorted sublists.
Bucket sort
An efficient, versatile data type sorting algorithm that partitions a data range into buckets, sorts each indivdual bucket using an efficient sorting algorithm, and then concatenates the sorted buckets.
Heap sort
An efficient, in-place sorting algorithm that builds a heap of elements from the list, and then repeatedly extracts and places the maximum element from the heap at the end of the sorted list.
IntrospectiveSort
IntroSort or introspective sort is a hybrid sorting algorithm that combines the strengths of quick sort and heap sort. It offers efficient sorting performance in most cases while maintaining a worst-case time complexity of O(n log n).
This book will change your understanding of sorting algorithms forever and you will be able to crack interview questions related to sorting algorithms.
Buy NowCustomer Reviews
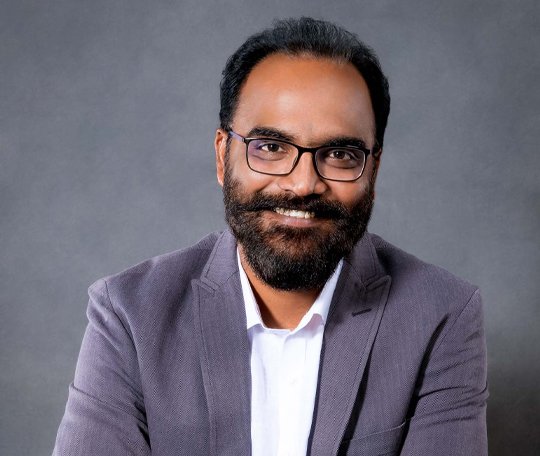