Count and Say
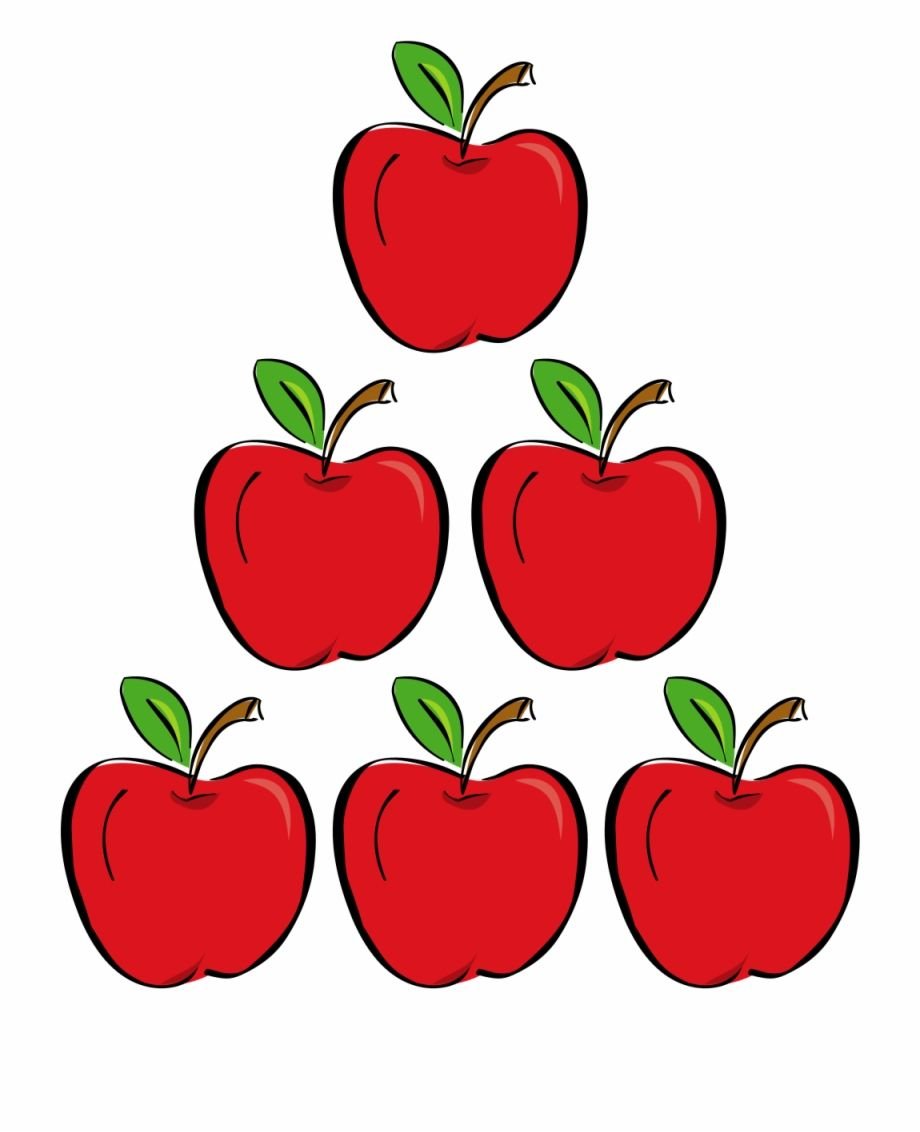
Problem: Count and Say
Problem Definition:
The "Count and Say" sequence generates subsequent terms by describing the previous term in a certain way. Each term is obtained by "saying" the digits of the previous term.
Sequence Definition:
- The sequence begins with "1".
- Subsequent terms describe the previous term, count the occurrences of digits, and concatenate the count with the digit itself.
- For instance, "1" is "one 1" = "11", "11" is "two 1s" = "21", and so on.
Objective:
To write a function countAndSay(n) that returns the nth term of the "Count and Say" sequence.
Approach:
- The function follows a recursive approach where each term is computed based on the description of the previous term.
- It iterates through the previous term, counts the consecutive occurrences of each digit, and generates the next term accordingly.
- For instance, given the input n = 4, the function generates the term by describing the previous term countAndSay(n - 1).
Execution Example (For n = 4):
- Base case: countAndSay(1) = "1"
- countAndSay(2) describes "1" as "one 1" = "11"
- countAndSay(3) describes "11" as "two 1s" = "21"
- countAndSay(4) describes "21" as "one 2, one 1" = "12" + "11" = "1211"
Functionality:
The countAndSay(n) function handles the base case (n=1) and computes subsequent terms by describing the previous term digit-wise.
Algorithm:
- Initialize the base case: countAndSay(1) = "1".
- For n > 1, use the previous term to generate the next term by counting consecutive occurrences of each digit.
Implementation:
-
Define the function countAndSay(n) in a way that:
- For n = 1, return "1" (base case).
- For n > 1, iterate through the previous term, count consecutive occurrences of each digit, and generate the next term accordingly.
This breakdown provides a clear understanding of the problem, the sequence's logic, and the approach to implement the countAndSay(n) function to generate terms in the "Count and Say" sequence.
Python Code
class Solution:
def countAndSay(self, n: int) -> str:
# Base case: If n is 1, return the base sequence "1"
if n == 1:
return "1"
# Recursively call countAndSay for (n - 1)
prev = self.countAndSay(n - 1)
result = "" # Initialize the result string
count = 1 # Initialize count of consecutive digits
# Loop through the previous sequence to generate the current sequence
for i in range(1, len(prev)):
# Compare current digit with the previous one
if prev[i] == prev[i - 1]:
# If the current digit is the same as the previous one, increase the count
count += 1
else:
# If it's different, append the count and the digit to the result, then reset the count
result += str(count) + prev[i - 1]
count = 1 # Reset count for the new digit
# Add the count and the last digit after the loop ends
result += str(count) + prev[-1]
return result # Return the generated sequence
# Test cases
solution = Solution()
# Take input from user for n
n = int(input())
# Generate sequence for input n and print the result
output = solution.countAndSay(n)
print(output)
Explanation :
- Class Definition: There's a class named 'Solution' containing a method 'countAndSay'.
- countAndSay Method:
- This method takes an integer 'n' as input and generates the Count and Say sequence for that value.
- It employs recursion. If 'n' is 1, it returns the base sequence, which is simply "1".
- Otherwise, it recursively calls 'countAndSay' for 'n - 1' and processes the sequence to generate the next sequence.
- It iterates through the previous sequence, counting consecutive digits and appending the count and digit to the result.
- The final generated sequence is returned.
- Test Cases:
- The script then creates an instance of 'Solution'.
- It prompts the user to enter a value for 'n'.
- It generates the Count and Say sequence for the input 'n' using the 'countAndSay' method and prints the result.
This algorithm works by describing the digits of the previous sequence in terms of the count of consecutive identical digits. For instance, for 'n=4', the sequence starts as "1", then it reads as "one 1", so it becomes "11", and so on.
Java Code
import java.util.Scanner;
class Solution {
// Method to generate the Count and Say sequence for a given n
public String countAndSay(int n) {
// Base case: If n is 1, return the base sequence "1"
if (n == 1) {
return "1";
}
// Recursively call countAndSay for (n - 1) to get the previous sequence
String prev = countAndSay(n - 1);
StringBuilder result = new StringBuilder(); // StringBuilder to store the result
int count = 1; // Initialize count of consecutive digits
// Loop through the previous sequence to generate the current sequence
for (int i = 1; i < prev.length(); i++) {
// Compare current digit with the previous one
if (prev.charAt(i) == prev.charAt(i - 1)) {
count++; // If the current digit is the same as the previous one, increase the count
} else {
// If it's different, append the count and the digit to the result, then reset the count
result.append(count).append(prev.charAt(i - 1));
count = 1; // Reset count for the new digit
}
}
// Add the count and the last digit after the loop ends
result.append(count).append(prev.charAt(prev.length() - 1));
return result.toString(); // Return the generated sequence
}
public static void main(String[] args) {
Solution solution = new Solution();
Scanner scanner = new Scanner(System.in);
// System.out.print("Enter a value for n: ");
int n = scanner.nextInt();
// Generate the Count and Say sequence for input n and print the result
String output = solution.countAndSay(n);
System.out.println(output);
scanner.close();
}
}
Explanation :
- Class Definition: There's a class named 'Solution' containing two main parts: the countAndSay method and the 'main' method.
- countAndSay Method:
- This method takes an integer 'n' as input and generates the Count and Say sequence for that value.
- It uses recursion. If 'n' is 1, it returns the base sequence, which is simply "1".
- Otherwise, it recursively calls 'countAndSay' for 'n - 1' and processes the sequence to generate the next sequence.
- It iterates through the previous sequence, counting consecutive digits and appending the count and digit to the result.
- The final generated sequence is returned as a string.
- main Method:
- It creates an instance of 'Solution'.
- It uses a 'Scanner' to receive user input for the value of 'n'.
- Then it generates the Count and Say sequence for the input 'n' using the 'countAndSay' method.
- Finally, it prints the result.
This algorithm describes the digits of the previous sequence in terms of the count of consecutive identical digits. For example, for n=4, the sequence starts as "1", then it reads as "one 1", so it becomes "11", and so on. The program takes the user's input for n and generates the corresponding sequence.
C++ Code
#include <iostream>
#include <string>
using namespace std;
class Solution {
public:
// Function to generate the Count and Say sequence for a given n
string countAndSay(int n) {
// Base case: if n is 1, return the base sequence "1"
if (n == 1) {
return "1";
}
// Recursively call countAndSay for (n - 1)
string prev = countAndSay(n - 1);
string result = ""; // Initialize the result string
int count = 1; // Initialize count of consecutive digits
// Loop through the previous sequence to generate the current sequence
for (int i = 1; i < prev.size(); ++i) {
// Compare current digit with the previous one
if (prev[i] == prev[i - 1]) {
// If the current digit is the same as the previous one, increase the count
count++;
} else {
// If it's different, append the count and the digit to the result, then reset the count
result += to_string(count) + prev[i - 1];
count = 1; // Reset count for the new digit
}
}
// Add the count and the last digit after the loop ends
result += to_string(count) + prev[prev.size() - 1];
return result;
}
};
int main() {
Solution solution;
int n;
// cout << "Enter a value for n: ";
cin >> n;
// Generate the Count and Say sequence for input n and print the result
string output = solution.countAndSay(n);
cout << output << endl;
return 0;
}
Explanation :
- Class Definition: The class 'Solution' contains one method.
- countAndSay Method:
- This method takes an integer 'n' as input and generates the Count and Say sequence for that value.
- It's a recursive method.
- If 'n' is 1, it returns the base sequence, which is simply "1".
- Otherwise, it recursively calls 'countAndSay' for n - 1 and stores the result in 'prev'.
- It iterates through the previous sequence, counting consecutive digits and appending the count and digit to the 'result' string.
- Finally, it returns the generated sequence as a string.
- main Function:
- It creates an instance of 'Solution'.
- It uses cout to prompt the user to enter a value for 'n'.
- It reads the value of n from the user using 'cin'.
- Then it generates the Count and Say sequence for the input ;n' using the 'countAndSay' method.
- Finally, it prints the generated sequence using 'cout'.
The "Count and Say" sequence reads as follows: Starting with "1", the subsequent sequences describe the previous sequence in terms of the count of consecutive identical digits. For example, for n = 4, the sequence starts as "1", then it reads as "one 1", so it becomes "11", and so on. The program takes the user's input for n and generates the corresponding sequence.
Advantages:
- Simple Logic: The algorithm's logic is straightforward, making it easy to understand and implement.
- Efficient Space Complexity: It generates sequences without storing all previous sequences, resulting in efficient space usage.
- Linear Time Complexity: It has a linear time complexity relative to the length of the generated sequence, making it relatively efficient for sequence generation.
Disadvantages:
- Performance for Large Inputs: As the input value n grows larger, the time complexity increases linearly, potentially impacting performance for very large values of n.
- Inefficiency in Retrieving Specific Sequences: If you need to retrieve sequences at random positions rather than sequentially, this algorithm may not be the most efficient.
Real World Applications:
- String Compression: Used in various data compression algorithms where sequences need to be represented efficiently.
- Encoding and Decoding: Applied in encoding schemes where sequences need to be represented in a concise manner.
- Pattern Recognition: In some cases, this algorithm's pattern-generating nature finds application in certain pattern recognition tasks.
Test Cases:
Test Case 1: Input:
5
Output:
111221
Explanation:
- The program prompts the user to input a value for
n
, which is5
. - The
countAndSay
method of theSolution
class is called with the input value5
. - The method generates the sequence by recursively calling itself for
n - 1
, which is4
. - For
n = 4
, the previous sequence is"1211"
. - The method loops through the previous sequence and counts consecutive digits. It generates the current sequence
"111221"
. - The generated sequence
"111221"
is printed as the output.
Test Case 2: Input:
6
Output:
312211
Explanation:
- The program prompts the user to input a value for
n
, which is6
. - The
countAndSay
method of theSolution
class is called with the input value6
. - The method generates the sequence by recursively calling itself for
n - 1
, which is5
. - For
n = 5
, the previous sequence is"111221"
. - The method loops through the previous sequence and counts consecutive digits. It generates the current sequence
"312211"
. - The generated sequence
"312211"
is printed as the output.
Summary:
The "Count and Say" algorithm is a simple yet effective way to generate sequences based on the count of consecutive digits in the previous sequence. Its straightforward logic and efficient space complexity make it suitable for various applications requiring sequence generation or compression. However, it might face performance challenges for very large inputs and might not be the optimal choice for accessing specific sequences randomly due to its sequential nature. Despite this, it finds utility in various fields, including data compression, encoding schemes, and pattern recognition tasks.