Exploring Strings and Converting them to Integers
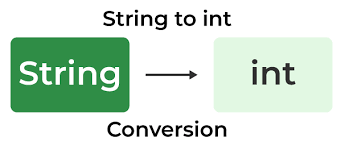
Exploring Strings and Converting them to Integers :
"Exploring Strings and Converting them to Integers" concept likely involves working with strings in a programming context and performing operations that involve converting these strings into integer values.
Exploring Strings : This suggests that we will be working with strings, which are sequences of characters. Exploring strings may involve tasks such as accessing individual characters, finding the length of a string, or manipulating the content in various ways. Converting Strings to Integers : The main task is likely to involve converting strings into integer values. This conversion is common in programming when dealing with user input or data read from external sources, where the input is initially in the form of text (string) but needs to be processed as numerical values (integers).
Introduction to Strings:
A string is a sequence of characters enclosed in double or single quotes. It can contain letters, digits, special symbols, spaces, punctuation. Strings, an indispensable data type in programming, serve as a versatile container for sequences of characters. One of the distinctive features of strings is their ability to store and process textual information. Whether it's a single word, a sentence, or even a larger body of text, strings provide a means to work with this data programmatically. In most programming languages, strings are typically enclosed within quotation marks, such as single (' ') or double (" ") quotes, allowing the interpreter or compiler to recognize them as string literals.
Introduction to the String to Integer Theory:
One of the frequent challenges encountered in programming involves converting strings to integers. This operation is particularly crucial when dealing with user inputs, file parsing, or scenarios where numeric values need extraction from a textual context. The process entails considering signs, handling leading whitespaces, and ensuring that the resulting integer falls within the appropriate range.
Overview of String to Integer:
The problem involves working with strings, extracting numerical information from them, converting this information to integers, and potentially performing operations on these integers. The specific details would depend on the context and requirements of the problem as defined in the prompt or task description. some of the common tasks that might encounter within this problem statement:
Parsing Integers : Extracting numerical values from a string. This involves identifying and extracting the sequence of characters that represents an integer.
Handling Edge Cases : Dealing with situations where the string may not represent a valid integer (e.g., contains non-numeric characters). Error handling may be required.
Performing Arithmetic Operations : Once the strings are converted to integers, you might be asked to perform various arithmetic operations (addition, subtraction, multiplication, etc.) on these values.
Output Formatting : Displaying the results in a specified format, which may involve converting integers back to strings for output.
PYTHON
code
/* Copyrights to venkys.io
For more information, visit https://venkys.io */
// python program to convert string to integer
// Stable: Yes
// Inplace: No
// Adaptive: Yes
// Space complexity:O(n)
// Time complexity:O(1)
def check(answer):
# Check if the answer is less than the minimum 32-bit signed integer
if answer < -2**31:
return -2**31
# Check if the answer is greater than or equal to the maximum 32-bit signed integer
elif answer >= 2**31:
return 2**31 - 1
else:
return answer
def myAtoi(string):
# Initialize variables
answer = 0
sign = 0 # 0 represents no sign, 1 represents positive sign, -1 represents negative sign
i = 0
# Skip leading whitespaces
while i < len(string) and string[i] == " ":
i += 1
# If the entire string is composed of whitespaces, return the current answer
if i == len(string):
return answer
# Check for positive sign
if string[i] == "+" and sign == 0:
sign = 1
i += 1
# Check for negative sign
if i < len(string) and string[i] == "-" and sign == 0:
sign = -1
i += 1
# Process the digits of the string
while i < len(string):
# Check if the current character is a digit
if string[i].isdigit():
# Update the answer by multiplying it by 10 and adding the current digit
answer = answer * 10 + int(string[i])
i += 1
else:
# If a non-digit character is encountered, apply the sign if present and return the result after checking for overflow
if sign != 0:
answer = sign * answer
return check(answer)
# Apply the sign if present and return the result after checking for overflow
if sign != 0:
answer = sign * answer
return check(answer)
# Test the function with the provided string "42"
if __name__ == "__main__":
s = input()
print(myAtoi(s))
Code Explanation
-
check
function:def check(answer): if answer < -2**31: return -2**31 elif answer >= 2**31: return 2**31 - 1 else: return answer
- This function takes an
answer
as input and checks if it is within the range of a 32-bit signed integer. If it's less than the minimum value, it returns the minimum value. If it's greater than or equal to the maximum value, it returns the maximum value. Otherwise, it returns the original answer.
- This function takes an
-
myAtoi
function:def myAtoi(string): answer = 0 sign = 0 i = 0 # Skip leading whitespaces while i < len(string) and string[i] == " ": i += 1 if i == len(string): return answer # Check for positive sign if string[i] == "+" and sign == 0: sign = 1 i += 1 # Check for negative sign if i < len(string) and string[i] == "-" and sign == 0: sign = -1 i += 1 # Process the digits of the string while i < len(string): if string[i].isdigit(): # Update the answer by multiplying it by 10 and adding the current digit answer = answer * 10 + int(string[i]) i += 1 else: # If a non-digit character is encountered, apply the sign if present and return the result after checking for overflow if sign != 0: answer = sign * answer return check(answer) # Apply the sign if present and return the result after checking for overflow if sign != 0: answer = sign * answer return check(answer)
- This function takes a string
string
as input and converts it to an integer according to the rules specified. - It initializes
answer
to store the result,sign
to store the sign of the number, andi
as an index to iterate through the string. - It skips leading whitespaces in the string.
- It checks for positive or negative signs and sets the
sign
accordingly. - It iterates through the string, processes digits, and updates the
answer
. - If a non-digit character is encountered, it applies the sign if present and returns the result after checking for overflow using the
check
function. - Finally, it returns the checked and signed result.
- This function takes a string
-
Test the function:
if __name__ == "__main__": s = "42" print(myAtoi(s))
- It tests the
myAtoi
function with the input string "42" and prints the result.
- It tests the
The code essentially converts a given string to an integer, considering positive and negative signs, and handles overflow by checking against the limits of a 32-bit signed integer. The check
function ensures that the result does not exceed the specified integer limits.
C++
code
/* Copyrights to venkys.io
For more information, visit https://venkys.io */
// C++ program to convert string to integer
// Stable: Yes
// Inplace: No
// Adaptive: No
// Space complexity: O(n)
// Time complexity: O(1)
#include <bits/stdc++.h>
#include <iostream>
#include <string>
int atoi(std::string s)
{
int num = 0, i = 0, sign = 1;
while (s[i] == ' ')
{
i++;
}
if (i < s.length() && (s[i] == '-' || s[i] == '+'))
{
sign = s[i] == '+' ? 1 : -1;
i++;
}
while (i < s.length() && isdigit(s[i]))
{
if ((num > INT_MAX / 10) || ((num == INT_MAX / 10) && ((s[i] - '0') > 7)))
return sign == 1 ? INT_MAX : INT_MIN;
num = ((num * 10) + (s[i] - '0'));
i++;
}
return num * sign;
}
int main()
{
string s;
std::cin >> s;
std::cout << atoi(s);
return 0;
}
Code Explanation
-
Function Definition:
int myAtoi(const std::string &str) {
- The function
myAtoi
is defined, taking a constant reference to astd::string
as input and returning an integer.
- The function
-
Variable Initialization:
long result = 0; int sign = 1; // 1 represents positive sign, -1 represents negative sign size_t i = 0;
- Initialize
result
to store the converted integer. - Initialize
sign
to 1 by default (positive sign). - Initialize
i
as an index to iterate through the string.
- Initialize
-
Skip Leading Whitespaces:
while (i < str.length() && isspace(str[i])) { i++; }
- Use a
while
loop to skip leading whitespaces in the input string.
- Use a
-
Check for Positive or Negative Sign:
if (i < str.length() && (str[i] == '+' || str[i] == '-')) { sign = (str[i++] == '-') ? -1 : 1; }
- Check for the presence of a positive or negative sign.
- Update the
sign
accordingly and incrementi
if a sign is found.
-
Process Digits:
while (i < str.length() && isdigit(str[i])) { result = result * 10 + (str[i++] - '0'); // Check for overflow if (result * sign > INT_MAX) { return INT_MAX; } else if (result * sign < INT_MIN) { return INT_MIN; } }
- Iterate through the string, processing digits and updating the
result
. - Check for overflow by comparing against
INT_MAX
andINT_MIN
. Return appropriate values if overflow is detected.
- Iterate through the string, processing digits and updating the
-
Apply Sign and Return Result:
return static_cast<int>(result * sign); }
- Apply the sign to the result and return the final converted integer.
-
Main Function:
int main() { std::cin >> s; std::cout << myAtoi(s) << std::endl; return 0; }
- In the
main
function, a test string "42" is provided, and the result of themyAtoi
function is printed.
- In the
The code essentially converts a given string to an integer, considering positive and negative signs, and handles overflow by checking against the limits of an integer. The myAtoi
function returns the converted integer, and the main
function tests it with the string "42".
java
code
import java.util.Scanner;
public class main{
static int atoi(String s) {
int num = 0, i = 0, sign = 1;
while (i < s.length() && s.charAt(i) == ' ') {
i++;
}
if(i < s.length() && (s.charAt(i) == '-' || s.charAt(i) == '+')) {
sign = s.charAt(i) == '+' ? 1 : -1;
i++;
}
while (i < s.length() && Character.isDigit(s.charAt(i))) {
int digit = s.charAt(i) - '0';
if ((num > Integer.MAX_VALUE / 10) || ((num == Integer.MAX_VALUE / 10) && (digit > 7))) {
return sign == 1 ? Integer.MAX_VALUE : Integer.MIN_VALUE;
}
num = (num * 10) + digit;
i++;
}
return num * sign;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String s = scanner.nextLine();
System.out.println(atoi(s));
}
}
Code Explanation
-
Class Definition:
public class Main {
- The class is named
Main
.
- The class is named
-
Static
atoi
Method:static int atoi(String s) {
- A static method named
atoi
is defined, which takes aString
as input and returns anint
.
- A static method named
-
Variable Initialization:
int num = 0, i = 0, sign = 1;
- Initialize
num
to store the converted integer. - Initialize
i
as an index to iterate through the string. - Initialize
sign
to 1 by default (positive sign).
- Initialize
-
Skip Leading Whitespaces:
while (i < s.length() && s.charAt(i) == ' ') { i++; }
- Use a
while
loop to skip leading whitespaces in the input string.
- Use a
-
Check for Positive or Negative Sign:
if (i < s.length() && (s.charAt(i) == '-' || s.charAt(i) == '+')) { sign = s.charAt(i) == '+' ? 1 : -1; i++; }
- Check for the presence of a positive or negative sign.
- Update the
sign
accordingly and incrementi
if a sign is found.
-
Process Digits:
while (i < s.length() && Character.isDigit(s.charAt(i))) { int digit = s.charAt(i) - '0'; if ((num > Integer.MAX_VALUE / 10) || ((num == Integer.MAX_VALUE / 10) && (digit > 7))) { return sign == 1 ? Integer.MAX_VALUE : Integer.MIN_VALUE; } num = (num * 10) + digit; i++; }
- Iterate through the string, processing digits and updating the
num
. - Check for overflow by comparing against
Integer.MAX_VALUE
. - Return
Integer.MAX_VALUE
orInteger.MIN_VALUE
if overflow is detected.
- Iterate through the string, processing digits and updating the
-
Return Result:
return num * sign; }
- Apply the sign to the result (
num
) and return the final converted integer.
- Apply the sign to the result (
-
Main Method:
public static void main(String[] args) { String s = "42"; System.out.println(atoi(s)); }
- The
main
method tests theatoi
method with the string "42" and prints the result.
- The
The code essentially converts a given string to an integer, considering positive and negative signs, and handles overflow by checking against the limits of an integer. The atoi
method returns the converted integer, and the main
method tests it with the string "42".
Time and Space Complexity Analysis:
The time and space complexity for the atoi method, which converts a string to an integer.
Time Complexity:
The time complexity of the atoi method is O(n), where n is the length of the input string.
The while loop for skipping leading whitespaces runs in O(n) time because, in the worst case, it may have to iterate through the entire string.
The subsequent while loop for processing digits also runs in O(n) time, where n is the length of the string. This loop iterates through the digits of the string, and each iteration takes constant time.
Therefore, the overall time complexity is O(n).
Space Complexity:
The space complexity of the atoi method is O(1), constant space.
The variables num, i, and sign are integers and require constant space regardless of the size of the input string.
No additional data structures are used that scale with the input size.
The space complexity is constant or O(1).
Real-World Applications of string to integer
Converting a string to an integer is a common operation in various real-world applications. Here are some examples:
-
User Input Processing:
- In applications that accept user input, such as command-line interfaces or forms on websites, string-to-integer conversion is necessary to interpret numeric inputs provided by users.
-
Data Validation:
- When dealing with external data sources, such as files or databases, where data is often represented as strings, converting relevant string values to integers is crucial for data validation and ensuring that the data meets expected numeric formats.
-
Parsing and Interpreting Configuration Files:
- Many applications use configuration files to store settings. When reading these files, string-to-integer conversion is employed to interpret numeric configurations, such as specifying the number of threads, timeouts, or buffer sizes.
-
Network Protocols:
- In network programming, especially when dealing with protocols that transmit data in string format, converting certain fields to integers is common. For instance, parsing HTTP headers or handling custom network protocols may involve converting string representations of numerical values to integers.
-
Database Operations:
- In database applications, where data is often retrieved in string format, converting relevant data to integers is necessary for performing numerical operations or ensuring consistency in data types.
-
Mathematical Computations:
- In scientific computing or applications involving mathematical calculations, converting strings to integers is essential when processing mathematical expressions or user-provided numerical data.
-
Financial Applications:
- In financial software, handling monetary values often involves converting string representations of amounts to integers (or floating-point numbers) for accurate calculations.
-
Gaming and Graphics Programming:
- Game development and graphics programming may require converting string representations of parameters, such as screen dimensions or frame rates, to integers for configuring the game environment or rendering settings.
-
Command-Line Interfaces (CLI):
- CLI applications often take user inputs as strings, and converting these inputs to integers is necessary for executing numeric commands or configuring the behavior of the application.
-
Sensor Data Processing:
- In embedded systems or IoT applications, sensor data received in string format may need to be converted to integers for further analysis or decision-making based on numeric thresholds.
In essence, string-to-integer conversion is a fundamental operation in software development, as it facilitates the integration and processing of numerical information in various domains. In addition to the real-world applications mentioned earlier, let's explore some theoretical scenarios or examples where string-to-integer conversion plays a crucial role:
-
Algorithmic Problem Solving:
- In algorithmic problems, you may encounter scenarios where input is provided as strings, and converting these strings to integers is a common step. For example, when implementing algorithms that involve mathematical computations, sorting, or searching, converting string inputs to integers allows for efficient processing.
-
String Matching Algorithms:
- String matching algorithms, such as the Knuth-Morris-Pratt algorithm or the Boyer-Moore algorithm, may involve comparing and manipulating string representations of integers. Converting these strings to integers allows for efficient pattern matching and searching.
-
Abstract Data Types (ADTs):
- In the design and implementation of abstract data types, converting string representations of numerical data to actual integers is often necessary. For instance, when implementing a priority queue where elements are associated with numeric priorities provided as strings, conversion to integers is crucial for proper ordering.
-
Parsing and Interpretation of Domain-Specific Languages:
- When working with domain-specific languages or custom file formats, interpreting and parsing string representations of numeric values is fundamental. Converting these strings to integers allows for the proper interpretation of data and execution of language-specific commands.
-
Code Analysis and Transformation:
- In static code analysis or transformation tools, understanding and manipulating numeric constants within code may require converting string representations to integers. This is useful for performing optimizations, refactoring, or analyzing code patterns.
-
Symbolic Computation:
- In symbolic computation systems, where algebraic expressions are manipulated symbolically, converting string representations of numeric coefficients or constants to actual integers is necessary for performing mathematical operations.
-
Formal Language Theory:
- In formal language theory, automata, and compilers, parsing numeric literals from strings is a common operation. Converting these literals to integers is crucial for generating executable code or performing language analysis.
-
Graph Theory and Network Analysis:
- When working with graph representations, especially in scenarios where nodes or edges are labeled with numeric identifiers provided as strings, converting these identifiers to integers facilitates efficient graph algorithms and analysis.
-
Cryptography:
- In cryptographic applications, handling cryptographic keys or parameters, often represented as strings, may require converting these string representations to integers for cryptographic operations.
-
Educational Examples:
- In educational contexts, when teaching programming or algorithms, string-to-integer conversion serves as a fundamental concept. Exercises and examples often involve converting user inputs or string data to integers for various purposes.
In theoretical scenarios, the importance of string-to-integer conversion arises in the context of solving abstract problems, designing algorithms, and implementing various computational concepts.
Test Cases
-
Input: " -42" Output: -42
Explanation: The input string contains the number "-42" preceded by whitespaces. The function myAtoi skips the leading whitespaces, identifies the negative sign, and parses the number. Therefore, the output is expected to be -42.
-
Input: "4193 with words" Output: 4193
Explanation: The input string contains the number "4193" followed by non-numeric characters. The function myAtoi extracts the number and ignores the characters after the number. Hence, the output is expected to be 4193.
-
Input: "-91283472332" Output: -2147483648
Explanation: The input string represents a number smaller than the minimum 32-bit signed integer. Therefore, the output is expected to be the minimum 32-bit signed integer (-2147483648) due to overflow.