How to think like a programmer
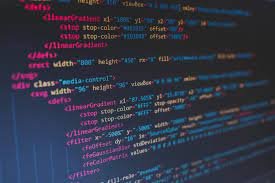
How to think like a programmer - an introduction
Introduction
Well, coding is making the logic understandable to a computer system.
A thorough understanding of the problem is the first step towards solving a problem.
This series of steps of understanding a problem becomes the first step of writing an algorithm.
Let us take a problem statement: You must perform the "Sum of the Integer numbers starting from 1000 to 10,000."
What is the first thing you perform as described above?
Understand the problem
-
What data is given in the problem statement?
-
What is the operation that is asked for?
-
Are there any boundary conditions given? (upper limit or lower limit)
Once the data is gathered from the problem statement, you can now proceed with writing an algorithm.
What data is given in the problem statement?
Integers
What is the operation that is asked for?
Sum
Are there any boundary conditions given? (upper limit or lower limit)
Yes,
lower-limit is 1000
Upper limit is 10000.
Algorithm
-
Assign two integer variable lower_limit = 1000 and upper_limit=10000.
-
Declare a variable called ‘Sum’ to hold the final result.
-
Initialize the ‘Sum’ to lower_limit, Sum = lower_limit = 1000;
-
Add integer ‘1’ everytime to the lower limit and add it to the ‘Sum’ until the lower_limit is less than or equal to upper_limit
-
lower_limit = lower_limit + 1;
-
Sum = lower_limit + 1;
With this algorithm you can start coding in your favorite programming language.
Python Code
lower_limit = 1000
upper_limit = 10000
Sum = 0
for i in range(lower_limit, upper_limit):
lower_limit=lower_limit+1
Sum=lower_limit+Sum
print Sum
C++ Code
int main()
{
int lower_limit = 1000;
int upper_limit = 10000;
int sum = 0;
for (;lower_limit <= upper_limit;lower_limit++)
sum = lower_limit + sum;
cout << sum;
return 0;
}
Summary
Now you have learnt how to understand a problem, understand the data given, and then translate the problem into an algorithm.
Once the algorithm has been written, you can code in the language of your choice. Python and C++ were chosen in the above example.
Hope you have learnt the art and science of how to think like a programmer.