REMOVE VOWELS FROM A STRING
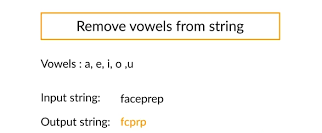
REMOVE VOWELS FROM A STRING
Brief Description :
The program "remove vowels from a string" is designed to take a input string and remove all the vowels (i.e., 'a', 'e', 'i', 'o', 'u') from it. The program scans the input string character by character, and if a character is not a vowel, it adds it to a new string. The resulting string contains the original input string with all the vowels removed. This program can be implemented in various programming languages such as Python, Java, C++, and others using different methods such as iteration, regular expressions, or built-in string manipulation functions.
Advantages And Disadvantages:
Advantages:
- Simplification of Text: It can help simplify text for certain applications where vowels are not necessary, such as in specific types of encoding or data processing.
- Input Validation: It can be used as a part of input validation processes in software development to ensure that input strings meet certain criteria.
- Language Processing: It can be used in natural language processing tasks where the presence of vowels is not relevant to the analysis being performed.
Disadvantages:
- Loss of Meaning: Removing vowels from a string can lead to a loss of meaningful information, especially in natural language contexts, as vowels are integral to understanding and pronunciation.
- Cultural or Linguistic Considerations: In some languages and cultural contexts, removing vowels may not be appropriate or may lead to misinterpretation of the text.
- Overgeneralization: The process of removing vowels may oversimplify text data, potentially distorting the original meaning or making it less readable.
Algorithm:
Step 1: Start Step 2: Accept the input string from the user Step 3: Create an empty string to store the result (e.g., resultString = "") Step 4: For each character, char, in the input string:
- If char is not a vowel (i.e., 'a', 'e', 'i', 'o', 'u', both uppercase and lowercase versions):
- Append char to the resultString Step 5: End of loop Step 6: Display or return the resultString as the output Step 7: Stop
Explanation:
- Start: The program begins.
- Accept the Input: User provides the input string.
- Initialize Empty String: Create an empty string called resultString to store the characters that are not vowels.
- Iterate Through Input String: For each character in the input string, check if it's a vowel. If it's not a vowel, add it to the resultString.
- End of Loop: Repeat the process for each character in the input string.
- Display or Return Output: Once all characters have been processed, display or return the resultString as the output.
- Stop: The program ends.
This algorithm efficiently removes vowels from the input string without modifying the original string. It handles both uppercase and lowercase vowels and provides the resulting string as output.
Program in Python:
def remove_vowels(input_string): return ''.join(char for char in input_string if char.lower() not in 'aeiou')
def count_vowels(input_string): return sum(1 for char in input_string if char.lower() in 'aeiou')
if name == "main": input_string = input()
result_removed_vowels = remove_vowels(input_string)
count_original_vowels = count_vowels(input_string)
print(result_removed_vowels) # print String after removing vowels
print(count_original_vowels) # print Number of vowels in the original string
# Program in c++:
---
#include <iostream>
#include <string>
std::string removeVowels(const std::string& inputString) {
std::string result;
for (char ch : inputString) {
if (tolower(ch) != 'a' && tolower(ch) != 'e' && tolower(ch) != 'i' && tolower(ch) != 'o' && tolower(ch) != 'u') {
result += ch;
}
}
return result;
}
int main() {
std::string inputString;
// std::cout << "Enter a string: ";
std::getline(std::cin, inputString);
std::string result = removeVowels(inputString);
std::cout << result << std::endl;
return 0;
}
Program in JAVA:
import java.util.Scanner;
public class Main {
//Function to remove vowels from the given String
public static String VSDRemoveVowels(String s) {
String output="";
for(int i=0;i<s.length();i++) {
if(s.charAt(i)=='A'||s.charAt(i)=='a'||
s.charAt(i)=='E'||s.charAt(i)=='e'||
s.charAt(i)=='I'||s.charAt(i)=='i'||
s.charAt(i)=='O'||s.charAt(i)=='o'||
s.charAt(i)=='U'||s.charAt(i)=='u') {
continue;
}
else {
output+=s.charAt(i);
}
}
return output;
}
//Function to print output
public static void VSDprintOutput(String out) {
System.out.println(out);
}
public static void main(String args[]) {
Scanner scanner = new Scanner(System.in);
String s = scanner.nextLine();
String out=VSDRemoveVowels(s);
VSDprintOutput(out);
}
}
Real World Scenarios:
The task of removing vowels from a string can be applied to various real-world scenarios:
- Data Cleaning: In data preprocessing for text analysis, removing vowels can be a step in preparing the text for further analysis.
- Text Processing: In some natural language processing applications, removing vowels from text might be useful for specific purposes like creating a simplified version of the text or for encoding/decoding purposes.
- Data Security: In certain encryption algorithms or data obfuscation techniques, removing vowels from a string could be one step in the process of securing sensitive information.
- User Input Validation: In software development, removing vowels from a user's input can be used as a form of input validation or data sanitization to ensure that certain strings meet specific criteria.
- Language Learning Tools: In language learning or educational applications, removing vowels from a string could be part of exercises designed to help learners recognize and process consonants in words.
Test Cases
-
INPUT : "Hello, World!"
OUTPUT :
"Hll, Wrld!"
3The function removes vowels from the input string by iterating over each character and keeping only those that are not vowels.
For the input "Hello, World!": 'H', 'l', 'l', ',', ' ', 'W', 'r', 'l', 'd', and '!' are kept. 'e', 'o', and 'o' are vowels, so they are removed. The resulting string is "Hll, Wrld!". The next function counts the number of vowels in the input string by iterating over each character and counting those that are vowels.
For the input "Hello, World!": 'e', 'o', and 'o' are vowels. The count of vowels in the original string is 3.
-
INPUT : "AEIOUaeiou"
OUTPUT:
""
10The function removes vowels from the input string by iterating over each character and keeping only those that are not vowels.
For the input "AEIOUaeiou": There are no consonants (non-vowels) in the string. All characters are vowels ('A', 'E', 'I', 'O', 'U', 'a', 'e', 'i', 'o', 'u'), so they are all removed. The resulting string is an empty string (""). The next function counts the number of vowels in the input string by iterating over each character and counting those that are vowels.
For the input "AEIOUaeiou": All characters are vowels. The count of vowels in the original string is 10.
-
INPUT : "The quick brown fox jumps over the lazy dog."
OUTPUT :
"Th qck brwn fx jmps vr th lzy dg."
5The function removes vowels from the input string by iterating over each character and keeping only those that are not vowels.
For the input "The quick brown fox jumps over the lazy dog.": All characters except 'e', 'u', 'i', 'o', and 'o' (vowels) are kept. The resulting string is "Th qck brwn fx jmps vr th lzy dg.". The next function counts the number of vowels in the input string by iterating over each character and counting those that are vowels.
For the input "The quick brown fox jumps over the lazy dog.": 'e', 'u', 'i', 'o', and 'o' are vowels. The count of vowels in the original string is 5.