Strings - An Introduction
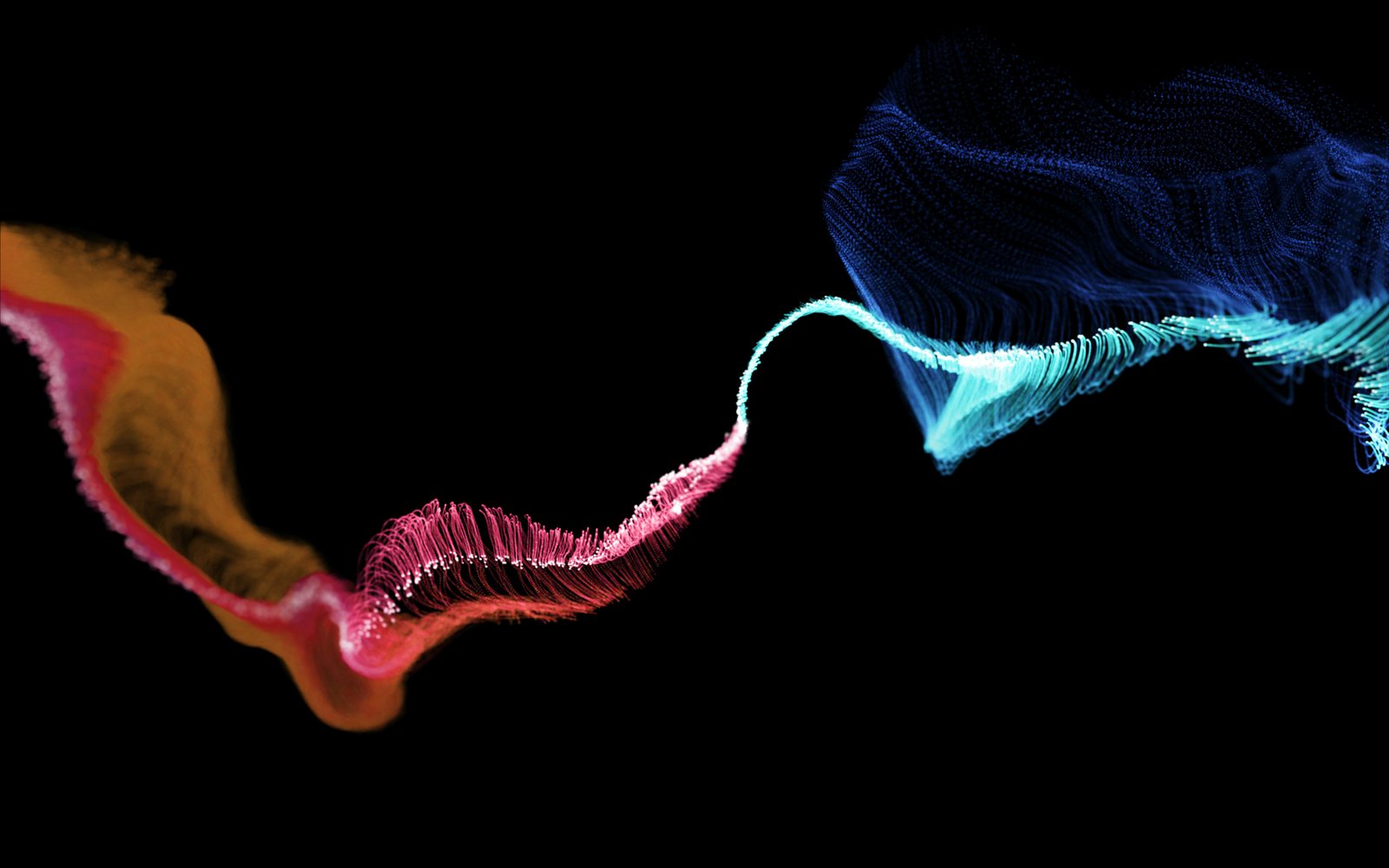
Strings Introduction
The initial use of a computer was to process numerical data but now they are regularly used to handle character or non-numerical data. This post discusses how this data is stored and operated. Word processing software is one of the leading applications of computers today. Generally, it entails pattern matching, testing for the occurrence of specific characters 'S' within a given text 'T'. As "word" has an alternative meaning in computer science, we often use "string" instead when talking about sequences of characters, hence phrases like "string processing", "string manipulation" or "text editing" as substitutes for "word processing".
Strings Basic Terms
A character set is used to communicate with the computer in each programming language.
Alphabet: A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
Digits: 0 1 2 3 4 5 6 7 8 9
Special characters: + − / * ( ) , . $ =
Strings are finite sequences of zero or more characters.
The number of characters in a string is known as its length.
An empty string or null string is a string with no characters.
Specific strings will be denoted by enclosing their characters in single quotation marks. Quotation marks will also serve as string delimiters.
Assume that S1 and S2 are strings. The string consisting of S1 followed by S2 is called the concatenation of S1 and S2. It will be denoted by S1//S2.
A string Y is called a substring of a string S if there are strings X and Z such that S = X//Y//Z
Using the above representation, If X is an empty string, then Y is called an initial substring of S, and if Z is an empty string then Y is called a terminal substring of S.
Strings Definition
Strings are one of the most basic data types in computer programming. A string is simply a sequence of characters. In most programming languages, there is no such thing as a "string data type" - instead, strings are usually represented as arrays of characters.
In C, for example, a string literal (i.e. a sequence of characters enclosed in double quotes) is actually an array of characters with a null character ('\0') added at the end to signify the end of the string. In other words, the following two declarations are equivalent:
char my_string[] = "This is a string";
char *my_string = "This is a string";
The second form is more commonly used since it doesn't require you to know the length of the string in advance (the null character at the end takes care of that).
String Processing
Strings are one of the most commonly used data types in programming, and as such, there are many different ways to process them. In this section, we'll take a look at some of the most common string processing methods.
One of the most basic things you can do with a string is to simply print it out. This can be done with the print() function in most programming languages:
print("Hello world!")
This will output the string "Hello world!" to the console.
If you want to save a string to a variable, you can do so using the assignment operator (=):
my_string = "Hello world!"
This will save the string "Hello world!" to the variable my_string. You can then access it later by using the variable name:
print(my_string) // outputs "Hello world!"
Another common operation is concatenation, which is when you combine two or more strings together. This is typically done using the + operator:
my_string = "Hello" + "world!" // outputs "Helloworld!"
C++ version of the program
#include <iostream>
#include <string>
int main() {
// Define a string variable
std::string message = "Hello, world!";
// Print the string to the console
std::cout << message << std::endl;
return 0;
}
Questions
Let W be the string(S) VSDEVELOPERS.
(a) Find the length of the given string S.
(b) List all substrings of S.
(c) List all the initial substrings of S.