WORD SEARCH
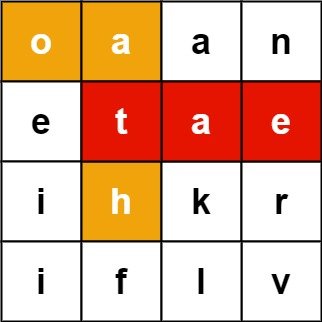
WORD SEARCH
INTRODUCTION
A word search program is a computer application or script designed to generate and solve word search puzzles. Word search puzzles are popular games that involve finding and circling words hidden within a grid of letters.
OVERVIEW OF WORD SEARCH
A word search is a popular word puzzle game that involves finding and circling specific words hidden within a grid of letters. The grid is typically square or rectangular, and the words can be placed horizontally, vertically, diagonally, forward, or backward. The objective is to locate all the words in the grid based on a list of given words.
Here's an overview of the key aspects of word searches:
- Components of a Word Search:
- Grid: A matrix of letters where the words are hidden.
- Words: A list of words that the player needs to find in the grid.
- Direction: Words can be placed in various directions—horizontally, vertically, diagonally, forward, or backward.
- Creating a Word Search:
- The process of creating a word search involves selecting words, determining their positions in the grid, and filling the remaining spaces with random letters.
- Designers often ensure that words do not overlap and are distributed in a balanced manner across the grid.
- Solving a Word Search:
- Players search for the words in the grid based on the given list.
- Words are usually circled or marked to indicate that they have been found.
- Some word searches have additional challenges, such as hidden themes or extra letters that form a message when read in order.
- Educational Applications:
- Word searches are widely used in education to reinforce vocabulary and spelling skills.
- They are suitable for various subjects, allowing educators to create puzzles related to specific topics or lessons.
- Entertainment and Relaxation:
- Word searches are commonly found in newspapers, magazines, puzzle books, and online platforms as a form of entertainment.
- Many people enjoy solving word searches for relaxation and as a leisure activity.
- Digital Word Searches:
- With the advent of technology, digital versions of word searches are prevalent in mobile apps, websites, and software.
- Digital word searches often offer features like dynamic puzzle generation, different difficulty levels, and interactive solving experiences.
- Customization and Themes:
- Word searches can be customized for specific occasions or themes. For example, holiday-themed word searches or puzzles related to a particular field (e.g., science, sports) are common.
- Themes can add an extra layer of interest and challenge to the puzzle.
- Variations:
- Some word searches have variations, such as crosswords combined with word search elements or word searches with hidden messages formed by unused letters.
Overall, word searches are versatile puzzles enjoyed by people of all ages. They serve as a valuable educational tool, a source of entertainment, and a relaxing pastime
Problem Statement:
You are tasked with creating a Python program that generates a word search puzzle. The program should take a list of words and create a grid where these words are hidden in various directions. Users should be able to interact with the program to find the hidden words.
#copyrights to venkys.io
#for more programs visit venkys.io
#python program for word search
import random
def generate_word_search(words, size):
# Create an empty grid
grid = [[' ' for _ in range(size)] for _ in range(size)]
# Place words randomly in the grid
for word in words:
direction = random.choice(['horizontal', 'vertical', 'diagonal'])
row, col = random.randint(0, size - 1), random.randint(0, size - 1)
if direction == 'horizontal':
if col + len(word) <= size:
for i in range(len(word)):
grid[row][col + i] = word[i]
elif direction == 'vertical':
if row + len(word) <= size:
for i in range(len(word)):
grid[row + i][col] = word[i]
elif direction == 'diagonal':
if row + len(word) <= size and col + len(word) <= size:
for i in range(len(word)):
grid[row + i][col + i] = word[i]
# Fill the remaining empty spaces with random letters
for i in range(size):
for j in range(size):
if grid[i][j] == ' ':
grid[i][j] = random.choice('ABCDEFGHIJKLMNOPQRSTUVWXYZ')
return grid
def display_word_search(grid):
for row in grid:
print(' '.join(row))
def word_search():
# print("Enter the words to include in the puzzle (separated by spaces):")
words = input().split()
# print("Enter the size of the puzzle grid:")
grid_size = int(input())
# print("\nWord Search Puzzle:")
word_search_grid = generate_word_search(words, grid_size)
display_word_search(word_search_grid)
# print("\nList of Words to Find:")
for word in words:
print(word)
if __name__ == "__main__":
word_search()
STEP-BY-STEP EXPLAINATION
- The random library is used for generating random values, crucial for placing words randomly in the grid.
- The generate_word_search function initializes an empty grid with spaces. The size of the grid is determined by the size parameter. It's a square grid.
- For each word in the list, the program randomly chooses a direction ('horizontal', 'vertical', or 'diagonal') and selects a random starting position (row,col) within the grid.
- Depending on the chosen direction, the program checks if the word can fit in that direction without going out of bounds. If it can, the program places the letters of the word in the corresponding positions in the grid.
- After placing the words, the program fills the remaining empty spaces in the grid with random letters. The function returns the final word search grid.
- The display_word_search function takes a grid as input and prints it row by row, separating each letter with a space .The word_search function initializes a list of words and a grid size. It generates a word search puzzle using the generate_word_search function, displays the puzzle using display_word_search , and prints the list of words to find.
- This block ensures that the word_search function is executed when the script is run as the main program.
Test Case 1: Null List of Words
- Input:
Enter the words to include in the puzzle (separated by spaces): Enter the size of the puzzle grid: 5
Explanation: In this case, an empty list of words is provided. The output should be an empty grid with random letters.
Test Case 2: True Word Search
- Input:
Enter the words to include in the puzzle (separated by spaces): PYTHON CODE SEARCH Enter the size of the puzzle grid: 10
Explanation: The input includes words "PYTHON," "CODE," and "SEARCH." The program should generate a word search grid containing these words in random directions.
Test Case 3: False Word Search
- Input:
Enter the words to include in the puzzle (separated by spaces): HELLO WORLD Enter the size of the puzzle grid: 3
Explanation: The grid size is too small to accommodate the words "HELLO" and "WORLD" in any direction. The output should be a grid with random letters.
//copyrights to venkys.io
// for more programs visit venkys.io
// java program for word search
import java.util.Arrays;
import java.util.Random;
import java.util.Scanner;
public class WordSearchGenerator {
public static char[][] generateWordSearch(String[] words, int size) {
char[][] grid = new char[size][size];
// Initialize the grid with spaces
for (char[] row : grid) {
Arrays.fill(row, ' ');
}
Random random = new Random();
// Place words randomly in the grid
for (String word : words) {
String direction = randomDirection(random);
int row = random.nextInt(size);
int col = random.nextInt(size);
if ("horizontal".equals(direction) && col + word.length() <= size) {
for (int i = 0; i < word.length(); i++) {
grid[row][col + i] = word.charAt(i);
}
} else if ("vertical".equals(direction) && row + word.length() <= size) {
for (int i = 0; i < word.length(); i++) {
grid[row + i][col] = word.charAt(i);
}
} else if ("diagonal".equals(direction) && row + word.length() <= size && col + word.length() <= size) {
for (int i = 0; i < word.length(); i++) {
grid[row + i][col + i] = word.charAt(i);
}
}
}
// Fill the remaining empty spaces with random letters
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
if (grid[i][j] == ' ') {
grid[i][j] = (char) ('A' + random.nextInt(26));
}
}
}
return grid;
}
private static String randomDirection(Random random) {
String[] directions = {"horizontal", "vertical", "diagonal"};
return directions[random.nextInt(directions.length)];
}
public static void displayWordSearch(char[][] grid) {
for (char[] row : grid) {
for (char cell : row) {
System.out.print(cell + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// System.out.print("Enter the words to include in the puzzle (separated by spaces): ");
String[] words = scanner.nextLine().split(" ");
// System.out.print("Enter the size of the puzzle grid: ");
int gridSize = scanner.nextInt();
// System.out.println("\nWord Search Puzzle:");
char[][] wordSearchGrid = generateWordSearch(words, gridSize);
displayWordSearch(wordSearchGrid);
// System.out.println("\nList of Words to Find:");
for (String word : words) {
System.out.println(word);
}
// Close the scanner
scanner.close();
}
}
STEP-BY-STEP EXPLAINATION
These statements import necessary classes for handling arrays and generating random numbers.
- generatewordsearch Method: Generates a word search puzzle. It initializes an empty grid, places words in different directions randomly, and fills the remaining empty spaces with random letters.
- randomDirection Method: Randomly selects a direction from the array of possible directions.
- displayWordsearch Method: Displays the generated word search grid.
- main Method: Initializes the list of words and grid size, generates the word search puzzle, displays it, and prints the list of words to find.
Test Case 1: Null List of Words
- Input:
Enter the words to include in the puzzle (separated by spaces): Enter the size of the puzzle grid: 5
Explanation: In this case, an empty list of words is provided. The output should be an empty grid with random letters.
Test Case 2: True Word Search
- Input:
Enter the words to include in the puzzle (separated by spaces): JAVA CODE SEARCH Enter the size of the puzzle grid: 10
Explanation: The input includes words "JAVA," "CODE," and "SEARCH." The program should generate a word search grid containing these words in random directions.
Test Case 3: False Word Search
- Input:
Enter the words to include in the puzzle (separated by spaces): HELLO WORLD Enter the size of the puzzle grid: 3
Explanation: The grid size is too small to accommodate the words "HELLO" and "WORLD" in any direction. The output should be a grid with random letters.
//copyrights to venkys.io
// for more programs visit venkys.io
// c++ program for word search
#include <iostream>
#include <vector>
#include <cstdlib>
#include <ctime>
std::vector<std::vector<char>> generateWordSearch(const std::vector<std::string>& words, int size) {
std::vector<std::vector<char>> grid(size, std::vector<char>(size, ' '));
// Initialize the grid with spaces
for (auto& row : grid) {
std::fill(row.begin(), row.end(), ' ');
}
// Seed for random number generation
std::srand(std::time(0));
// Place words randomly in the grid
for (const auto& word : words) {
std::string direction = randomDirection();
int row = std::rand() % size;
int col = std::rand() % size;
if (direction == "horizontal" && col + word.length() <= size) {
for (size_t i = 0; i < word.length(); i++) {
grid[row][col + i] = word[i];
}
} else if (direction == "vertical" && row + word.length() <= size) {
for (size_t i = 0; i < word.length(); i++) {
grid[row + i][col] = word[i];
}
} else if (direction == "diagonal" && row + word.length() <= size && col + word.length() <= size) {
for (size_t i = 0; i < word.length(); i++) {
grid[row + i][col + i] = word[i];
}
}
}
// Fill the remaining empty spaces with random letters
for (int i = 0; i < size; i++) {
for (int j = 0; j < size; j++) {
if (grid[i][j] == ' ') {
grid[i][j] = 'A' + std::rand() % 26;
}
}
}
return grid;
}
std::string randomDirection() {
std::vector<std::string> directions = {"horizontal", "vertical", "diagonal"};
return directions[std::rand() % directions.size()];
}
void displayWordSearch(const std::vector<std::vector<char>>& grid) {
for (const auto& row : grid) {
for (char cell : row) {
std::cout << cell << ' ';
}
std::cout << '\n';
}
}
int main() {
std::cout << "Enter the words to include in the puzzle (separated by spaces): ";
std::vector<std::string> words;
std::string input;
std::getline(std::cin, input);
std::istringstream iss(input);
std::string word;
while (iss >> word) {
words.push_back(word);
}
// std::cout << "Enter the size of the puzzle grid: ";
int gridSize;
std::cin >> gridSize;
// std::cout << "\nWord Search Puzzle:\n";
auto wordSearchGrid = generateWordSearch(words, gridSize);
displayWordSearch(wordSearchGrid);
// std::cout << "\nList of Words to Find:\n";
for (const auto& word : words) {
std::cout << word << '\n';
}
return 0;
}
STEP-BY-STEP EXPLAINATION
Header and Namespace:
These include statements provide access to necessary C++ libraries.
generateWordsearch Method:
This function generates a word search puzzle. It initializes a 2D vector (grid), randomly places words in different directions, and fills the remaining empty spaces with random letters.
displayWordsearch Function:
This function displays the generated word search grid.
main Function:
The main function initializes the list of words and grid size, generates the word search puzzle, displays it, and prints the list of words to find.
EXAMPLE FOR THE OUTPUT:
Word Search Puzzle: Z L P X G V G R O O M S Y Y J K R B E Y T C Z K G A J N Q N U K Q N R H E N A L T Y M Z D N T L L P U R S Y V X C M S Z S I F Z L E L O K H R T F L Y E Y R C U Z P N V X O H A V I T U Z C I O M Q O X S W N D J L G E S G H R U V H Y E X U H
List of Words to Find: PYTHON JAVA C HTML CSS JAVASCRIPT
Note that each time you run the program, the output will be different as the words are placed randomly in the grid.
Test Case 1: Null List of Words
- Input:
Enter the words to include in the puzzle (separated by spaces): Enter the size of the puzzle grid: 5
Explanation: In this case, an empty list of words is provided. The output should be an empty grid with random letters.
Test Case 2: True Word Search
- Input:
Enter the words to include in the puzzle (separated by spaces): C++ CODE SEARCH Enter the size of the puzzle grid: 8
Explanation: The input includes words "C++," "CODE," and "SEARCH." The program should generate a word search grid containing these words in random directions
Test Case 3: False Word Search
- Input:
Enter the words to include in the puzzle (separated by spaces): PROGRAMMING PUZZLE Enter the size of the puzzle grid: 4
Explanation: The grid size is too small to accommodate the words "PROGRAMMING" and "PUZZLE" in any direction. The output should be a grid with random letters.
TIME AND SPACE COMPLEXITY
TIME COMPLEXITY:
- Word Placement:
- For each word in the list, the program randomly selects a direction and a starting position in the grid. This operation is done for each word independently.
- Time complexity for placing each word is O(L), where L is the length of the word.
- Grid Filling:
- Filling the remaining empty spaces in the grid with random letters has a time complexity of O(N), where N is the total number of cells in the grid.
- Overall:
- If we have W words and a grid of size N x N, the overall time complexity is approximately O(W * L + N^2).
SPACE COMPLEXITY:
- Grid Storage:
- The program uses a 2D vector to represent the grid. The space complexity for storing the grid is O(N^2).
- Random Number Generation:
- The program uses random number generation for selecting directions and positions. The space complexity for this is typically O(1).
- Overall:
- The overall space complexity is O(N^2).
Here, W is the number of words, L is the average length of words, and N is the size of the grid.
REAL WORLD APPLICATIONS OF WORD SEARCH
- Education:
- Vocabulary Building: Word search puzzles are used in language education to reinforce and expand students' vocabulary. Teachers can create puzzles related to specific topics or subject areas.
- Spelling Practice: Word searches provide a fun way for students to practice spelling by identifying and locating words within a grid.
- Recreation and Entertainment:
- Magazines and Newspapers: Many newspapers and magazines feature word search puzzles as a recreational activity for readers. They provide a leisurely and engaging experience.
- Books: Puzzle books and collections often include word search puzzles alongside other types of puzzles.
- Cognitive Training:
- Brain Health: Word search puzzles, along with other types of word games, are often recommended for brain health and cognitive training. They can help improve memory, attention, and pattern recognition skills.
- Mobile Apps and Games:
- Mobile Games: Numerous mobile apps and games are dedicated to word search puzzles. These apps allow users to solve puzzles on their smartphones and tablets, offering a convenient and portable form of entertainment.
- Online Platforms:
- Websites and Online Platforms: Various websites and online platforms provide word search puzzles for users to solve. These can be themed, timed, or generated dynamically.
- Teaching and Training:
- Training Materials: Word search puzzles are incorporated into training materials for various professions. For example, medical students might use word searches related to medical terminology.