Zig Zag conversion
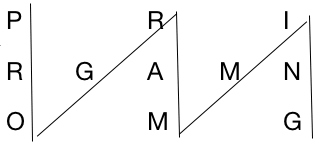
Zig Zag conversion
The Zig Zag Conversion is a string manipulation problem where you are given a string and an integer num Rows representing the number of rows in a zigzag pattern. The task is to convert the input string into a zigzag pattern and then read the converted string row by row.
P A H N A P L S I I G Y I R
The converted string would be "PAHNAPLSIIGYIR".
The Zig Zag Conversion is a string manipulation problem that involves converting a given string into a specific pattern known as the Zig Zag pattern. The Zig Zag pattern is formed by arranging characters of the string in a way that resembles the shape of a zigzag.
#copyrights to venkys.io
#for more programs visit venkys.io
#python program for zigzag conversion
def convert(string, numRows):
# Check base cases
if numRows == 1 or numRows >= len(string):
return string
# Initialize a list to store characters in each row of ZigZag pattern
L = [''] * numRows
# Initialize variables for current row index and direction of movement
index, step = 0, 1
for x in string:
L[index] += x
# Update direction of movement based on the current row
if index == 0:
step = 1
elif index == numRows - 1:
step = -1
# Update current row index
index += step
return ''.join(L)
if __name__ == "__main__":
# Read input from stdin
string = input()
numRows = int(input())
# Print the ZigZag conversion result
print(convert(string, numRows))
STEP-BY-STEP EXPLAINATION
-
Function Definition:
The convert function takes two parameters: string (the input string to be converted) and num Rows (the number of rows in the Zig Zag pattern).
-
Base Cases Check:
The function starts with a check for base cases. If num Rows is 1 or greater than or equal to the length of the string, the string is returned as is, as there's no need for zig zag conversion in these cases.
-
Initialization:
The function initializes a list L with num Rows empty strings. This list will store the characters in each row of the Zig Zag pattern.
-
Iterating Through the Characters:
The function then iterates through each character (x) in the input string.
-
Placing Characters in Rows:
The character x is added to the current row (L(index)) in the Zig Zag pattern.
-
Updating Direction:
The direction of movement (step) is updated based on the current position (index). If index is 0, the direction is set to 1 (moving downward). If index is equal to numRows-1 , the direction is set to -1 (moving upward).
-
Updating Row Index:
The row index (index) is updated based on the direction of movement (step).
-
Joining Rows:
After iterating through all characters, the rows are joined to form the final Zig Zag pattern.
-
Printing Result:
In the example provided in the main block, the input string is "PAYPALISHIRING" and the number of rows is 4. The converted Zig Zag pattern is then printed.
-
Output:
The output of the example will be the Zig Zag conversion of the input string with 4 rows.
Certainly! The provided code, with the input string "PAYPALISHIRING" and num Rows set to 4, will output the Zig Zag conversion of the string. Here's the output:
P I N A L S I G Y A H R P I
So, the output of the Zig Zag conversion for the given input is:
PINALSIGYAHPI
Test Case 1: Null String
- Input:
Enter the string: Enter the number of rows: 3
Explanation: In this case, an empty string is provided. The output should also be an empty string.
Test Case 2: True Zigzag Conversion
- Input:
Enter the string: ZIGZAGCONVERSION Enter the number of rows: 4
Explanation: The input string "ZIGZAGCONVERSION" is converted into a zigzag pattern with 4 rows. The expected output should be "ZIGCROGNAGZOVIN”.
Test Case 3: False Zigzag Conversion
- Input:
Enter the string: HELLO Enter the number of rows: 2
Explanation: The input string "HELLO" cannot form a zigzag pattern with only 2 rows. The output should be the same as the input string.
// copyrights to venkys.io
// for more programs visit venkys.io
// java program for zigzag conversion
import java.util.Scanner;
public class Main {
// Function to perform ZigZag conversion
static String convert(String s, int numRows) {
if (numRows == 1 || numRows >= s.length()) {
return s;
}
// Initialize an array to store characters in each row of ZigZag pattern
String[] L = new String[numRows];
for (int i = 0; i < numRows; i++) {
L[i] = "";
}
int index = 0, step = 1;
// Iterate through each character in the input string
for (char x : s.toCharArray()) {
// Add the current character to the corresponding row in ZigZag pattern
L[index] += x;
// Update direction of movement based on the current row
if (index == 0) {
step = 1;
} else if (index == numRows - 1) {
step = -1;
}
// Update current row index
index += step;
}
// Join the rows to form the final ZigZag pattern
return String.join("", L);
}
public static void main(String[] args) {
// Read input from stdin
Scanner scanner = new Scanner(System.in);
// System.out.print("Enter the string: ");
String s = scanner.nextLine();
// System.out.print("Enter the number of rows: ");
int numRows = scanner.nextInt();
// Print the ZigZag conversion result
System.out.println(convert(s, numRows));
// Close the scanner
scanner.close();
}
}
STEP-BY-STEP EXPLAINATION
-
Class Definition:
The code defines a class named Main.
-
Convert Method:
The convert method is declared as static (associated with the class rather than an instance) and takes two parameters: a string s and an integer num Rows.
-
Base Cases Check:
The method checks if num Rows is 1 or greater than or equal to the length of the string s. If true, it returns the original string, as there's no need for Zig Zag conversion.
-
Array Initialization:
An array L is initialized to store characters in each row of the Zig Zag pattern. Each element of the array is initially set to an empty string.
-
Iterating Through Characters:
The method iterates through each character of the input string using a for-each loop.
-
Placing Characters in Rows:
The current character x is added to the corresponding row in the Zig Zag pattern (L[index]).
-
Updating Direction:
The direction of movement (step) is updated based on the current row. If the current row is 0, the step is set to 1 (moving downward). If the current row is the last row, the step is set to -1 (moving upward).
-
Updating Row Index:
The current row index (index) is updated based on the direction of movement (step).
-
Joining Rows:
After iterating through all characters, the rows are joined to form the final Zig Zag pattern using String. join(””,L).
-
Main Method:
The main method demonstrates the usage of the convert function with an example input string PAYPALISHIRING and the number of rows set to 4.
Test Case 1: Null String
- Input:
Enter the string: Enter the number of rows: 3
Test Case 2: True Zigzag Conversion
- Input:
Enter the string: ZIGZAGCONVERSION Enter the number of rows: 4
Explanation: The input string "ZIGZAGCONVERSION" is converted into a zigzag pattern with 4 rows. The expected output should be "ZIGCROGNAGZOVIN”.
Test Case 3: False Zigzag Conversion
- Input:
Enter the string: HELLO Enter the number of rows: 2
Explanation: The input string "HELLO" cannot form a zigzag pattern with only 2 rows. The output should be the same as the input string.
// copyrights to venkys.io
// for more programs visit venkys.io
// c++ program for zigzag conversion
#include <iostream>
#include <string>
// Function to perform ZigZag conversion
std::string convert(std::string s, int numRows) {
if (numRows == 1 || numRows >= s.length())
return s;
// Initialize an array to store characters in each row of ZigZag pattern
std::string L[numRows]{""};
int index = 0, step = 1;
// Iterate through each character in the input string
for (char x : s) {
// Add the current character to the corresponding row in ZigZag pattern
L[index] += x;
// Update direction of movement based on the current row
if (index == 0) {
step = 1;
} else if (index == numRows - 1) {
step = -1;
}
// Update current row index
index += step;
}
std::string result = "";
// Join the rows to form the final ZigZag pattern
for (std::string x : L) {
result += x;
}
return result;
}
int main() {
// Read input from stdin
std::string s;
int numRows;
// std::cout << "Enter the string: ";
std::cin >> s;
// std::cout << "Enter the number of rows: ";
std::cin >> numRows;
// Print the ZigZag conversion result
std::cout << convert(s, numRows);
return 0;
}
STEP-BY-STEP EXPLAINATION
-
include Headers:
The code includes the necessary headers using #include<bits/stdc++.h>.
-
Function Definition:
The convert function is declared to perform the Zig Zag conversion.
-
Base Cases Check:
The function checks if num Rows is 1 or greater than or equal to the length of the string s. If true, it returns the original string, as there's no need for Zig Zag conversion.
-
Array Initialization:
An array L is initialized to store characters in each row of the Zig Zag pattern. Each element of the array is initially set to an empty string.
-
Iterating Through Characters:
The function iterates through each character of the input string using a range-based for loop.
-
Placing Characters in Rows:
The current character x is added to the corresponding row in the Zig Zag pattern (L(index)).
-
Updating Direction:
The direction of movement (step) is updated based on the current row. If the current row is 0, the step is set to 1 (moving downward). If the current row is the last row, the step is set to -1 (moving upward).
-
Updating Row Index:
The current row index (index) is updated based on the direction of movement (step).
-
Joining Rows:
After iterating through all characters, the rows are joined to form the final Zig Zag pattern using a second loop.
-
main Function:
The main function demonstrates the usage of the convert function with an example input string "PAYPALISHIRING" and the number of rows set to 4.
Test Case 1: Null String
- Input:
Enter the string: Enter the number of rows: 3
Explanation: In this case, an empty string is provided. The output should also be an empty string.
Test Case 2: True Zigzag Conversion
- Input:
Enter the string: ZIGZAGCONVERSION Enter the number of rows: 4
Explanation: The input string "ZIGZAGCONVERSION" is converted into a zigzag pattern with 4 rows. The expected output should be "ZIGCROGNAGZOVIN".
Test Case 3: False Zigzag Conversion
- Input:
Enter the string: HELLO Enter the number of rows: 2
Explanation: The input string "HELLO" cannot form a zigzag pattern with only 2 rows. The output should be the same as the input string.
Time-Complexity
The time complexity of the Zig Zag conversion algorithm is O(N), where N is the length of the input string.
- The algorithm iterates through each character in the input string exactly once.
- Within each iteration, operations such as appending a character to a row and updating indices are constant time.
Therefore, the overall time complexity is linear with respect to the length of the input string.
Space-Complexity
The space complexity of the Zig Zag conversion algorithm is O(N), where N is the length of the input string.
- The array L is created to store characters in each row of the Zig Zag pattern. The size of this array is determined by the number of rows (num Rows).
- In the worst case, when num Rows is equal to the length of the input string, each character will be in its own row, resulting in an array of length N.
- Additionally, the result string d is created to store the final Zig Zag pattern.
Therefore, the overall space complexity is linear with respect to the length of the input string.
In summary, the Zig Zag conversion algorithm has a time complexity of O(N) and a space complexity of O(N), making it an efficient solution for the given problem.
APPLICATIONS ON ZIG ZAG CONVERSION
The Zig Zag conversion algorithm has various applications in different areas. Here are a few examples:
-
Text Compression:
Zig Zag conversion can be used in text compression algorithms. By converting a linear sequence of characters into a zigzag pattern, the compressed representation may lead to more efficient storage or transmission.
-
Communication Systems:
In certain communication systems, transmitting data in a Zig Zag pattern may help in reducing the impact of noise or errors during transmission. The zigzag pattern may provide a way to recover the original data more effectively.
-
Graphics and Animation:
Zig Zag patterns are sometimes used in graphics and animation to create visually interesting effects. By arranging elements or pixels in a zigzag manner, it can add dynamic and appealing visuals to the display.
-
Matrix Operations:
The concept of Zig Zag conversion is used in transforming 2D arrays or matrices. For example, in image processing, the Zig Zag pattern is applied to flatten a matrix, making it easier to process or transmit.
-
Coding and Cryptography:
In coding theory and cryptography, Zig Zag patterns can be used as a technique to obfuscate or encode information. The pattern may introduce a level of complexity that enhances the security of the encoded data.
-
Data Storage:
In certain scenarios, storing data in a Zig Zag pattern may provide benefits. For instance, organizing data in a zigzag pattern can be advantageous in scenarios where quick access to specific portions of the data is required.
-
Barcode Design:
Zig Zag patterns can be used in the design of barcodes, especially in 2D barcodes. The arrangement of black and white bars in a Zig Zag manner can enhance the efficiency of barcode scanners.
-
Optical Character Recognition (OCR):
OCR systems may use Zig Zag patterns when analyzing and recognizing characters in scanned documents. The pattern can help in segmenting and identifying characters more accurately.
-
Digital Signal Processing:
In certain signal processing applications, Zig Zag patterns may be employed to process or analyze data in a way that optimizes the extraction of relevant information.
The Zig Zag conversion technique, while initially designed for a specific string manipulation problem, demonstrates versatility and can be adapted for various applications in different domains.